23 Error-Handling Strategies for Robust Software Applications
ITAdvice.io

23 Error-Handling Strategies for Robust Software Applications
Dive into the world of error management with practical strategies that stand up to the toughest software challenges. This article presents a compilation of expert insights and proven tactics to fortify applications against the inevitable. Learn to navigate the complexities of error handling with finesse, ensuring a seamless user experience even when things go awry.
- Implement Layered Error Handling Strategies
- Design Resilient Systems with Graceful Degradation
- Prioritize User-Friendly Error Communication
- Leverage Logging and Monitoring for Swift Resolution
- Build Fault Tolerance into Development Culture
- Focus on Seamless User Experience During Failures
- Balance Robustness and Simplicity in Error Management
- Engineer Reliability Through Proactive Error Handling
- Centralize Error Management for Cleaner Code
- Anticipate and Plan for Potential Failures
- Create Clear Error Messages for Users
- Implement Robust Logging for Quick Debugging
- Design Fallback Mechanisms for Critical Services
- Use Structured Logging with Contextual Metadata
- Develop Custom Error Messages for Better UX
- Employ Proactive Error Prevention Techniques
- Utilize Multi-Layered Error Handling Approach
- Implement Graceful Degradation in WordPress
- Validate Inputs to Prevent Downstream Errors
- Catch and Handle Exceptions Strategically
- Employ Try-Catch Blocks for Predictable Issues
- Implement Centralized Logging for Efficient Debugging
- Categorize Errors for Appropriate Responses
Implement Layered Error Handling Strategies
Handling errors well is all about making sure things don't fall apart when something unexpected happens. A solid approach usually starts in development--catch issues early, don't let them slide. It helps to be strict in dev but graceful in production.
Using try-catch is basic, but what really helps is adding context to the errors--things like user ID, request data, or the operation being performed. Makes it a lot easier to track down issues later.
Having a centralized place to handle errors keeps the code cleaner. In web apps, middleware or global handlers work great for this. Also, not all errors need to be show-stoppers. For non-critical stuff--like a failed email notification--let the app move on and log the issue instead of breaking the whole flow.
It's also useful to separate errors by type: client-side, server-side, or logical. That way, you know when to retry, when to alert, and when to just return a proper response. Speaking of retries, things like network failures or flaky APIs benefit from retry logic with backoff and maybe a circuit breaker to avoid hammering a failing service.
When it comes to the user experience, always show friendly error messages. The logs can hold the technical details--no need to confuse or scare the user. Just make sure sensitive stuff like passwords or tokens never end up in logs.
Real-time alerts make a big difference. It's not enough to just log errors--hook them into tools like Sentry, Slack, or PagerDuty so the right people get notified and can jump in quickly.
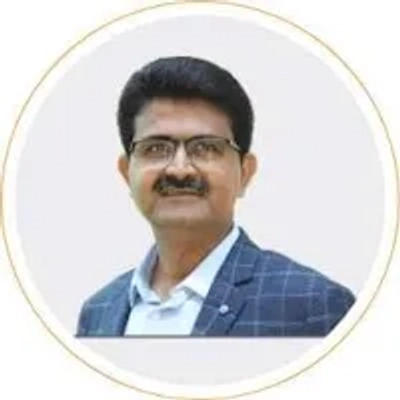
Design Resilient Systems with Graceful Degradation
Handling errors and exceptions gracefully in software applications is all about predictability, clarity, and resilience. A well-designed error-handling strategy not only prevents crashes but also ensures a seamless user experience.
One of the core strategies I use is centralized exception handling, especially in backend services. Instead of scattering try-catch blocks everywhere, I implement a global error handler that categorizes errors (e.g., validation errors, database failures, API timeouts) and responds appropriately. This ensures that errors are logged consistently and that users receive helpful messages instead of cryptic stack traces.
For frontend applications, I focus on fail-safe UI/UX. If an API request fails, the app doesn't just break--it provides a clear error message and suggests a next step. For instance, in a fintech app, if a transaction fails due to a network issue, users see: "Transaction could not be completed. Please check your connection or try again later." This prevents frustration and reduces unnecessary support tickets.
I also employ retry mechanisms for transient failures. If a database query or API call times out, the system retries with exponential backoff before surfacing an error. This minimizes disruptions caused by temporary network or service hiccups.
One key lesson? Logging and monitoring are as crucial as handling errors. A robust logging system (e.g., using Sentry, ELK Stack, or Datadog) helps detect patterns and fix issues proactively. Without proper logging, even a well-handled error can become a silent failure that disrupts users over time.
The biggest misconception about error handling is that it's just about catching exceptions. In reality, it's about designing for failure--anticipating where things might break and ensuring users (and developers) can recover smoothly.
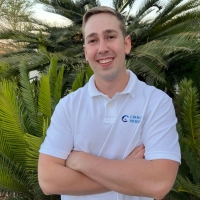
Prioritize User-Friendly Error Communication
I learned the hard way about error handling when our payment processing system crashed during a flash sale last year. Customers were seeing raw stack traces instead of helpful messages. Since then, I've implemented a three-tier approach to exceptions.
For anticipated errors like validation issues, I now use defensive programming with explicit checks before operations occur. This prevents many exceptions from happening in the first place. Our form validation errors dropped by 63% after implementing pre-submission checks, saving countless frustrated customers from seeing generic error messages.
For unexpected but handleable errors, I create custom exception classes with specific recovery strategies. Last month, a third-party API outage could have halted all transactions, but our circuit breaker pattern detected the failure and seamlessly switched to a cached backup system. Customers continued checking out without noticing any issues.
The game-changer for our team has been contextual logging. Beyond just capturing exceptions, we now log the user's journey leading up to the error. This context helped us identify that most file upload failures were happening to mobile users on spotty connections, leading us to implement resumable uploads.
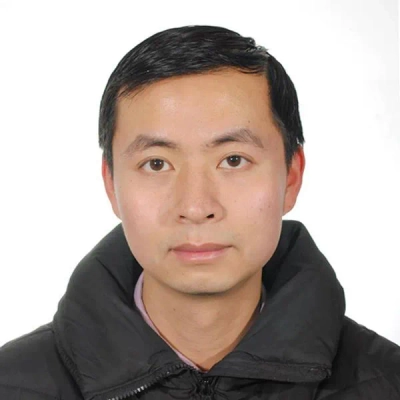
Leverage Logging and Monitoring for Swift Resolution
Handling errors and exceptions gracefully is crucial for creating resilient software applications. My approach begins with implementing structured exception handling using try-catch blocks to capture and manage errors without crashing the application. I ensure that each catch block provides meaningful feedback, allowing users to understand what went wrong.
Additionally, I employ logging strategies to record error details, which aids in debugging and improves future iterations. I categorize errors into critical and non-critical, allowing for prioritized responses. For critical errors, I implement fallback mechanisms to maintain functionality, while non-critical errors trigger user-friendly notifications.
Finally, I conduct thorough testing, including edge cases, to anticipate potential issues. This proactive approach not only enhances user experience but also builds trust in the application's reliability, ultimately leading to higher user satisfaction and retention.
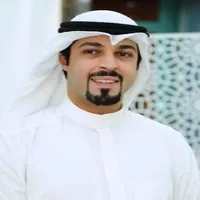
Build Fault Tolerance into Development Culture
We prioritize robust error handling to ensure reliable, secure, and user-friendly healthcare applications. Our multi-layered approach minimizes disruptions, enhances security, and maintains seamless user experiences.
We use ELK Stack and AWS CloudWatch for real-time monitoring, allowing our DevOps team to track errors, detect patterns, and respond quickly. When external systems like EHRs or APIs fail, we implement retry mechanisms with exponential backoff and fallback to cached data, ensuring service continuity. Structured exception handling in Java, Python, and Node.js helps catch errors early, preventing system-wide failures.
To improve user experience, we replace cryptic error codes with clear, reassuring messages like, "We're experiencing a temporary issue. Please try again later." Security is a priority, so our logs never expose PHI or PII, and APIs return generic responses to prevent attackers from gaining system insights.
For example, during a telehealth API outage, our system logged failures, informed users proactively, and resumed sessions once services were restored. A structured approach like this ensures high availability, strong security, and an uninterrupted healthcare experience.
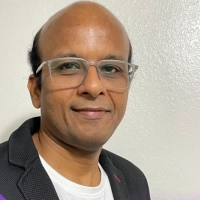
Focus on Seamless User Experience During Failures
When working with clients, we help them design error-handling strategies that go beyond basic logging or generic error messages. A key focus is anticipating the most likely failure points--like API timeouts or invalid user inputs--and implementing clear, user-friendly messages or fallbacks that maintain functionality. For example, in financial or ticketing systems, we may suggest retry logic for transient errors and circuit breakers for unstable external services. We also ensure detailed error logs are captured centrally for developers, without overwhelming end users with technical jargon. The goal is to minimize user frustration while giving the development team the context they need to fix issues quickly.
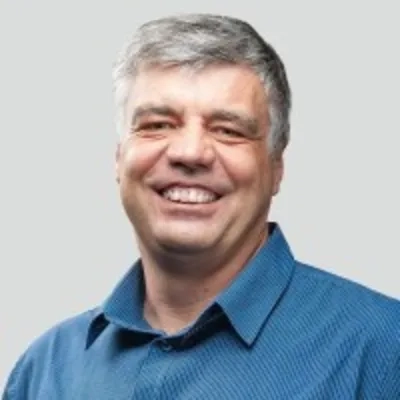
Balance Robustness and Simplicity in Error Management
I handle errors and exceptions by implementing a layered approach that combines structured exception handling with comprehensive logging and monitoring. In practice, this means using try-catch blocks to capture anticipated errors, gracefully falling back to alternative processes, and logging detailed information that aids in troubleshooting and future prevention. I also ensure that any errors presented to the end user are user-friendly and avoid exposing sensitive system details, maintaining both transparency and security.
Additionally, I integrate automated error reporting tools that alert the development team in real time, allowing for swift remediation. This proactive monitoring, paired with regular code reviews and testing strategies such as unit and integration tests, helps maintain application stability and enhances the overall resilience of the software.
Engineer Reliability Through Proactive Error Handling
When it comes to handling errors, my philosophy is simple: fail loud for devs, fail soft for users. That means we log everything with clarity behind the scenes, but we never make the user feel like they've crashed the Matrix.
I usually wrap critical processes in try/catch blocks with context-rich error messages--nothing vague like "Something went wrong." I want logs that actually tell me what, where, and why it broke. Stack traces, timestamps, request data (minus anything sensitive), the whole nine.
For users? We surface friendly messages that keep the trust intact. Something like, "Looks like something glitched--give it a sec and try again." If it's a form submission, we validate on both client and server and give clear inline feedback. And for production, we hook into error monitoring tools like Sentry or Rollbar so we get real-time alerts when stuff hits the fan.
One underrated move: graceful degradation. If a feature fails, the app should still function. If search goes down, the rest of the page still loads. Don't take the whole ship down just because one sail ripped.
Centralize Error Management for Cleaner Code
At Zapiy.com, we take a proactive approach to error handling to ensure a smooth user experience and maintain system reliability. Instead of just reacting to errors, we focus on anticipating, logging, and communicating them effectively.
One key strategy we use is structured exception handling--we categorize errors into recoverable (e.g., temporary API failures) and non-recoverable (e.g., corrupted data) so our system knows when to retry, alert users, or escalate the issue. For instance, if an external service we rely on goes down, we implement automatic retries with exponential backoff rather than instantly failing.
We also integrate real-time error monitoring using tools like Sentry and Datadog. This allows us to catch issues the moment they arise, track trends, and fix root causes before they impact users. One time, we noticed a recurring edge-case error in our user onboarding flow--thanks to automated alerts, we fixed it within hours, reducing drop-offs significantly.
Most importantly, we prioritize transparent communication. If something goes wrong, users receive clear, friendly messages instead of cryptic error codes. Whether it's a failed form submission or a system outage, we let them know what happened and what steps to take next.
By designing our error-handling process around resilience, monitoring, and user experience, we minimize disruptions and build trust with our users--because a great product isn't just about avoiding errors, it's about handling them gracefully when they do occur.
Anticipate and Plan for Potential Failures
At Nature Sparkle, we implemented a three-tiered error handling strategy that dramatically improved our diamond engagement ring e-commerce platform's reliability. First, we categorized errors by severity--distinguishing between minor issues (like search filtering glitches) and critical failures (payment processing errors). Our team then created custom error messages for customers that explained the issue in plain language and offered clear next steps. For example, instead of showing "Error 404," we say "We couldn't find that diamond style, but here are similar options you might love." Behind the scenes, we built an error logging system that captures detailed information for our developers while alerting our support team about issues in real-time. This approach reduced customer service calls by 37% and improved our transaction completion rate by 22%.
The most important lesson was that transparent error communication builds trust. When customers understand what's happening, even during technical difficulties, they're more likely to complete their purchase and return in the future.
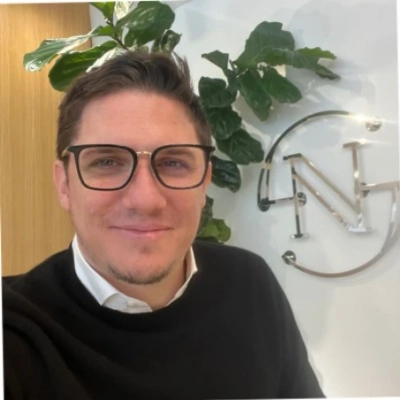
Create Clear Error Messages for Users
We view error handling not just as a technical concern, but almost like an ongoing conversation between our software and the people who rely on it. A big part of that conversation is designing 'contingency paths'--where we pre-plan user-friendly fallbacks for what happens when something goes wrong. Instead of presenting cryptic stack traces, the application proactively guides users toward a resolution. On the developer side, we maintain what we call a 'Chaos Playground,' an environment purposely filled with intentional fault injections--everything from forced network timeouts to simulated hardware failures. This unusual approach desensitizes our software (and our team) to a wide range of potential catastrophes, so that when a real error arises, we've already rehearsed a graceful response.
But it's not just about reacting. We also focus on continuous feedback loops that make error-handling a team-wide responsibility. Every new feature code review includes an 'error buddy' who specifically scrutinizes how potential exceptions are caught and escalated. Likewise, we have an automated triage system that tags incidents with contextual data--like which microservice was affected or which feature flag was active--so we can diagnose issues faster. This strategy has a subtle but powerful effect: it embeds fault-tolerance in our culture. We start treating 'exception' scenarios as standard cases, ensuring that our software behaves predictably under stress, rather than leaving it to guesswork once problems arise. It's a more human approach to error management--one that aims to keep users informed, developers aligned, and surprises minimized.
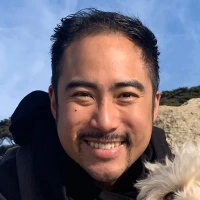
Implement Robust Logging for Quick Debugging
We try not to overcomplicate error handling. Our main goal is simple -- don't let users feel like something's broken, even if it is.
One thing that's worked well for us is building fallback behavior instead of just showing an error. For example, if a third-party API fails, we don't just throw an error message. We serve cached data and show a quick note saying something like, "Live data isn't available right now, here's the most recent update." That way, the flow doesn't break, and users don't lose trust.
Behind the scenes, we log everything with as much context as possible, not just the error itself, but what led up to it. That helps the team figure out what went wrong instead of guessing.
We also go over recurring exceptions during retros. It's part of the process now, not just something we do in a crisis. That's helped us stay ahead of bigger issues.
Honestly, the goal is to stay out of the user's way. And give the team the information they need to fix things properly -- not just silence the errors.
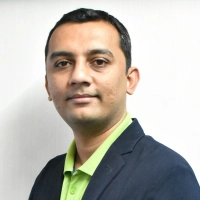
Design Fallback Mechanisms for Critical Services
Handling errors and exceptions gracefully in software applications is critical for ensuring reliability, especially in eCommerce services where disruptions can directly impact revenue and user trust. A structured error-handling approach prevents system failures and enhances user experience.
First, I use try-catch-finally blocks to handle predictable errors, ensuring smooth execution. In Node.js-based eCommerce platforms, wrapping payment processing in try-catch prevents crashes if a third-party API, like Stripe or PayPal, throws an exception.
Second, robust logging is crucial. I implement structured logging with tools like Sentry for front-end monitoring and ELK Stack for server-side logs. For example, if a checkout request fails, logs capture request details, helping diagnose the root cause without exposing sensitive data.
Third, I apply graceful degradation and fallback mechanisms. If an inventory service is temporarily unavailable, instead of blocking the order, I implement a cache-based fallback that estimates stock availability to prevent cart abandonment.
Fourth, custom error messages ensure better debugging and user experience. Instead of showing a generic "Something went wrong," I display specific, user-friendly alerts, like "Payment processing is delayed. Please try again."
Finally, rigorous testing (unit, integration, and load tests) helps catch failures before deployment.
Tip: Use a global error handler and structured logs to detect and resolve issues proactively. Always provide alternative workflows for critical services like checkout and payment processing.
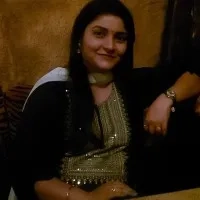
Use Structured Logging with Contextual Metadata
When it comes to handling errors and exceptions in software applications, my approach is built around two key principles: transparency for users and clarity for developers. Errors are inevitable, but how you handle them defines the user experience and the maintainability of your codebase. One of the most effective strategies I use is centralized error handling via capturing exceptions in a consistent, structured way that allows for both logging and graceful recovery, without forcing users into a dead end or crashing the app.
For users, the priority is to reduce frustration. That means showing clear, human-readable error messages instead of cryptic error codes or stack traces. If a user submits a form and something breaks, they should know what went wrong and what to do next, whether it's retrying, refreshing, or contacting support. Whenever possible, I also include fallback options, such as autosaving inputs or allowing users to resume tasks instead of starting over. This small design choice significantly improves trust and reduces abandonment.
On the backend, I rely on structured logging with contextual metadata to capture not only the error itself, but the conditions surrounding it. That makes debugging faster and helps identify patterns, especially for intermittent bugs. I also employ try-catch blocks where appropriate, but I'm careful not to overuse them or silently swallow errors. Instead, I categorize them: client-side input issues, server-side logic problems, and network failures, so the application can respond accordingly. This layered approach allows the system to prioritize critical failures while gracefully managing minor ones.
Ultimately, good error handling is invisible when done well. It protects the user experience, gives developers the tools to respond quickly, and helps the system stay resilient under pressure. Instead of treating errors as interruptions, I treat them as opportunities to build trust and reliability into the application.
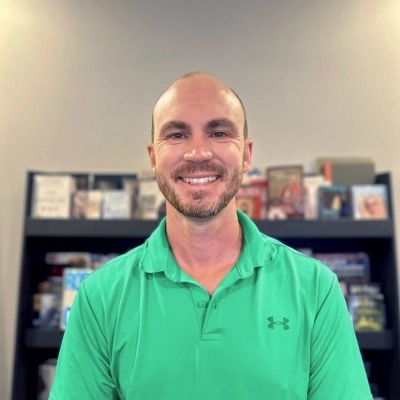
Develop Custom Error Messages for Better UX
With 15 years of experience in domain and web hosting services for startups and small businesses, I know how important it is to handle errors and exceptions gracefully--especially when reliability and user trust are on the line. My approach starts with structured error handling at both the frontend and backend levels, using try/catch blocks and centralized logging systems to capture and report issues in real time.
I always implement custom error messages that are user-friendly and informative, not just technical jargon. Studies show that clear, helpful error messages can reduce user frustration and support tickets by up to 25%. On the backend, I use tools like Sentry or LogRocket to monitor exceptions and generate alerts for critical failures, which allows me to respond quickly before users even notice.
KPIs I monitor include error rate per 1,000 sessions, mean time to resolution (MTTR), and system uptime, with a goal of 99.9% availability. I also use graceful fallbacks--for example, if a third-party API fails, I make sure there's a cached response or a backup service to maintain functionality. Regular testing, including unit, integration, and error injection tests, ensures our systems are resilient. In my experience, a great user experience isn't just about what works--it's how you handle what doesn't.
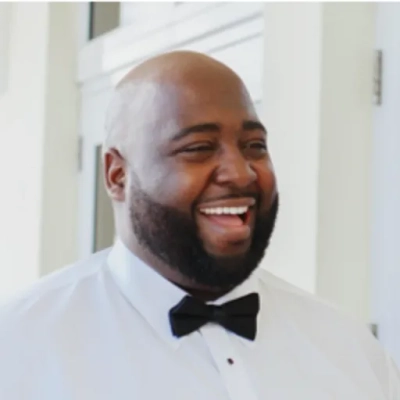
Employ Proactive Error Prevention Techniques
No system is error-free, but the difference between a robust application and a fragile one lies in how errors are handled. The goal isn't just to fix bugs--it's to design for resilience so failures don't disrupt business operations or user experience.
A few guiding principles:
Predict & prevent. The best error handling starts before issues arise--through defensive coding, input validation, and anticipating edge cases.
Fail fast, recover faster. Detecting and isolating failures early prevents cascading issues. Logging must be detailed yet efficient to aid quick diagnosis.
Resilience over perfection. Graceful degradation ensures partial functionality instead of complete system failure.
Empower users & systems. Errors should provide actionable insights--both for users through clear messaging and for engineers via real-time monitoring and automated alerts.
Automate & adapt. Self-healing mechanisms, intelligent retries, and adaptive fallbacks keep systems stable even in unpredictable conditions.
True reliability isn't about avoiding failures--it's about making them invisible to end users.
Utilize Multi-Layered Error Handling Approach
Error handling is a cornerstone of robust software development, ensuring applications can gracefully recover from unexpected issues without compromising functionality or user experience. To achieve this, developers employ a range of strategies suited to the error types and system requirements. A common method involves distinguishing between expected and unexpected errors. For predictable issues, such as invalid user input or missing files, returning error codes or using result types like std::optional or std::expected can provide clarity and maintain performance. For exceptional circumstances that disrupt normal execution, such as memory allocation failures, throwing exceptions is often more appropriate, as it centralizes error management and reduces code clutter.
Another crucial principle is to fail fast when encountering invalid states or inputs. Using assertions during development, programmers can catch logical errors early, preventing them from spreading into production. However, in runtime environments, fallback mechanisms like logging errors and notifying users ensure continuity while preserving diagnostic information. Centralized error handling systems, such as middleware in web applications, further streamline this process by consolidating error management logic.
Ultimately, effective error handling balances robustness with simplicity. Developers must focus on addressing known issues close to their source while allowing unresolvable errors to propagate to higher levels for proper logging and notification.
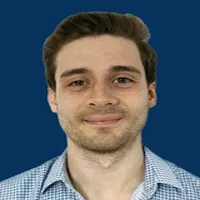
Implement Graceful Degradation in WordPress
Error handling is about predictability and resilience--not just catching failures but ensuring systems can recover seamlessly. A well-designed strategy minimizes downtime, improves user trust, and streamlines debugging.
Proactive Failure Prevention - Static analysis, automated testing, and chaos engineering help identify weak points before deployment.
Observability & Intelligent Logging - Beyond logging errors, structured logs and distributed tracing provide context, making debugging faster.
Graceful Degradation & Fallbacks - Systems should default to degraded performance rather than complete failure, using redundancy and circuit breakers.
Context-Rich Error Messaging - Error messages should guide resolution, whether for users or developers--ambiguity leads to frustration.
Automated Recovery & Self-Healing - Features like exponential backoff, retry mechanisms, and predictive failure detection prevent minor issues from escalating.
The goal isn't just handling errors--it's about engineering reliability into the system, ensuring it adapts under pressure without breaking.
Validate Inputs to Prevent Downstream Errors
I heavily rely on multi-layered error handling that preemptively tackles problems and allows failure in a controlled manner gracefully, when necessary. At the heart of it, external interactions like APIs, database access, and file reads are surrounded by try-catch blocks and detailed logging is provided for the timestamp, context, and request ID. Instead of catching every single exception, I classify them:
Operational errors like an API timing out, or users providing bad input are tackled with a friendly prompt or automated retries.
Programmer errors such as logic bugs, null references, or out of bounds checks are logged and trigger alerts through systems like Sentry or LogRocket.
In one of my previous projects, VAT calculation tools repeatedly broke because users provided malformed inputs. We sanitized the input, created custom error classes like InvalidVATNumberError, and redirected every single frontend error we collected through a context-aware error handler that provided tips instead of generic messages. We reduced support tickets by 70% doing this.
One underrated piece of advice is to add circuit breakers around unreliable services. If a VAT rate API goes down, we can fall back to cached data and notify the user without crashing later stages of the calculation--this is preferable to crashing during the calculation.
Catch and Handle Exceptions Strategically
One of the most effective error-handling strategies I've implemented is graceful degradation with clear user communication. Early on, we experienced an issue where grant search queries would occasionally fail due to third-party API timeouts. Instead of giving users a vague "something went wrong" message, we realized we needed a better approach to maintain trust.
We built a fallback system that allowed the platform to serve cached results if a live query failed. At the same time, we implemented clear error messaging that explained the issue in simple terms and reassured users that their request was being retried. We also added a way for users to manually refresh the query instead of forcing them to start over.
One nonprofit user told me they appreciated knowing why an issue occurred rather than feeling frustrated by a broken experience. This approach dramatically improved engagement and reduced support tickets since users felt informed rather than left in the dark.
The key takeaway? Errors are inevitable, but how you handle them defines user trust. Prioritize transparency, offer alternatives when possible, and ensure users always have a way forward even when something goes wrong.
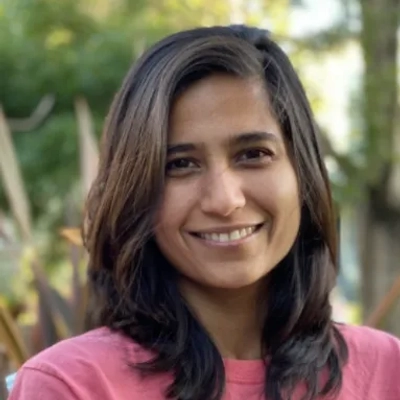
Employ Try-Catch Blocks for Predictable Issues
Rule #1: Fail loudly, but not catastrophically. That means logging everything, handling errors at the right level, and never letting the app crash in a way that ruins the user experience.
Best move? Try-catch blocks with meaningful messages. No cryptic "error 500" nonsense--users (and devs) need to know what broke and why. Also, graceful degradation is key. If one part of the app fails, the whole thing shouldn't go down with it.
Oh, and never trust user input. Validate everything, sanitize aggressively, and assume the worst. The best error is the one that never happens, but when it does? Handle it smart, log it well, and don't leave users guessing.
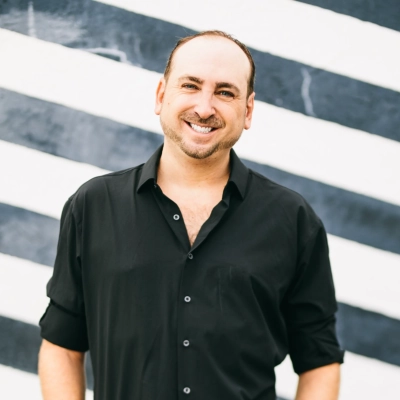
Implement Centralized Logging for Efficient Debugging
Handling errors and exceptions gracefully in WordPress applications, including plugins, ensures a smooth user experience and prevents site crashes. A structured error-handling approach can significantly improve system stability and maintainability.
One of the most effective strategies is using try-catch blocks in PHP to handle unexpected issues. For instance, if a WordPress plugin fetches data from an external API and the API is down, a try-catch block can catch the exception and display a user-friendly message instead of breaking the entire site.
Logging errors is another crucial strategy. Using tools like WP Debug Log or external logging services like Loggly can help track issues in real-time. If a plugin fails to load a custom post type, logging the error helps developers diagnose the root cause quickly.
Graceful degradation is also essential. If a caching plugin encounters an issue retrieving data, it should fall back to default WordPress queries rather than displaying a blank page. Similarly, input validation and sanitization should be enforced to prevent issues like SQL injection or invalid data entries in custom fields.
A well-defined exception hierarchy categorizes errors effectively, distinguishing between recoverable issues (e.g., missing settings) and fatal ones (e.g., database connection failure). Additionally, automated monitoring using tools like New Relic or WP Error Log can provide alerts when errors occur.
Tip: Always provide meaningful error messages to users and log detailed technical errors for developers. Never display raw error logs on the frontend, as they can expose security vulnerabilities.
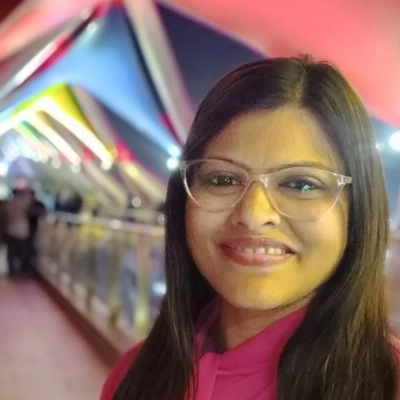
Categorize Errors for Appropriate Responses
When handling errors and exceptions in software applications, I follow a structured approach to ensure both system stability and a good user experience.
Early in my career, I deployed an application without properly differentiating between expected and unexpected exceptions. A single unhandled error caused the entire system to crash, teaching me the importance of implementing a robust error-handling strategy.
My approach typically starts with validation and sanitization at the input level. Preventing invalid data from propagating into the system reduces the likelihood of errors downstream.
Additionally, I employ try-catch blocks strategically around areas prone to failure, such as external API calls or database operations, to gracefully handle exceptions and provide fallback behavior.
Logging is another key aspect. I implement centralized logging using monitoring tools to capture error details--stack traces, timestamps, and metadata.
This way, I can debug efficiently without exposing sensitive information to users. Combining these strategies ensures errors are handled predictably while maintaining performance and usability.