10 Coding Practices for Cleaner and More Maintainable Code
Delve into the world of clean coding with expert-backed strategies that promise to elevate code maintainability and clarity. This article unpacks the essential practices that set apart novice programming from advanced craftsmanship. Discover the transformative power of principles like DRY, modularization, and clear documentation, as recommended by leading figures in software development.
- Merciless Modularization Transforms Code
- Follow the DRY Principle
- Write Clean, Self-Documenting Code
- Adhere to Single Responsibility Principle
- Use Consistent Naming and Documentation
- Adopt Modular Programming
- Emphasize Composability and Modularity
- Implement Single Responsibility Principle
- Write Small, Modular Functions
- Write Clear Comments and Small Functions
Merciless Modularization Transforms Code
The most transformational coding practice I've implemented is merciless modularization. By reducing large systems down into smaller, focused functions with clear responsibilities, I've significantly decreased cognitive burden and simplified troubleshooting.
Earlier in my career, I created monolithic code blocks that were difficult to maintain. Now, each function excels at one specific task. This approach allows me to quickly isolate and tackle problems without having to unravel large codebases. It's like using surgical accuracy rather than bulldozing through complex systems.
Practical implementation entails continuously asking oneself, "Can this be simplified?" "Does this function serve a single, clear purpose?" I've created a practice of writing self-documenting functions with meaningful variable names and minimal complexity.
This method has saved numerous hours of team collaboration, decreased possible bug surfaces, and made onboarding new devs much easier. The end result is simpler, more elegant code that scales efficiently and remains understandable even months after initial construction.
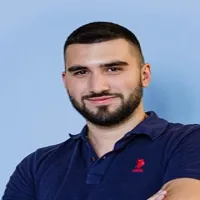
Follow the DRY Principle
One of the most valuable coding practices I've adopted for writing cleaner and more maintainable code is consistently following the DRY (Don't Repeat Yourself) principle. Early in my career, I found myself repeating similar logic across different parts of my codebase. It made things harder to maintain, especially when I needed to fix a bug or add a new feature.
By using the DRY principle, I started extracting reusable logic into functions, classes, or even separate modules. This helped me reduce duplication and keep my codebase much cleaner.
For example, if I had similar validation checks scattered throughout different areas of my app, I would refactor that into a single function that I could call whenever needed. Not only does this cut down on repetition, but it also makes it easier to apply changes—if there's a bug or improvement to be made in one piece of logic, I only need to fix it in one place.
This practice has greatly improved my development process. It saves time in the long run, as I spend less time fixing repetitive bugs, and it enhances collaboration because other developers can easily understand and work with my code.
It also makes my code much more adaptable to future changes or feature additions, since I can make adjustments in a centralized way rather than tracking down every occurrence of the same logic.
In short, adhering to DRY has made my code cleaner, more organized, and easier to maintain, which has been a game-changer for me.
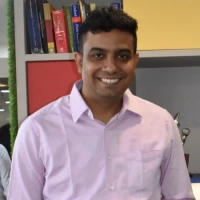
Write Clean, Self-Documenting Code
The most valuable coding practice I've adopted is writing clean, self-documenting code by prioritizing meaningful variable and function names, adhering to consistent formatting, and implementing SOLID principles. This practice significantly reduces reliance on excessive comments and ensures that the codebase communicates its intent clearly.
For example, instead of naming a function processData, I would name it filterExpiredSubscriptions to explicitly convey its purpose. Additionally, I strive to keep functions short, ensuring they do only one thing, and I write unit tests alongside the code to verify functionality early and often.
This practice has drastically improved our development process by reducing onboarding time for new developers, minimizing bugs, and streamlining code reviews. It has also fostered a collaborative environment where team members can easily understand and build upon each other's work, ultimately enhancing productivity and code maintainability.
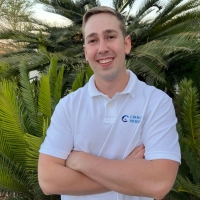
Adhere to Single Responsibility Principle
The most valuable coding practice I've adopted is writing small, modular functions with single responsibilities. By adhering to the Single Responsibility Principle (SRP), each function does one thing well, making the code easier to read, test, and maintain.
For example, instead of a single 100-line function handling user input, validation, and database insertion, I split it into three smaller functions. This separation improved clarity, allowed unit testing for each part, and made debugging faster since issues were isolated.
This practice has transformed my development process by reducing bugs, simplifying collaboration, and making future feature additions or refactors significantly easier. It's a game-changer for maintainable, scalable codebases.
Use Consistent Naming and Documentation
1. Follow consistent naming conventions
- Use descriptive, meaningful names for variables, functions, and classes
- Maintain consistent capitalization (e.g., camelCase for methods, PascalCase for classes)
- Avoid abbreviations unless they're widely understood in your domain
2. Write self-documenting code with clear structure
- Break down complex operations into well-named functions
- Keep functions focused on a single responsibility
- Use descriptive variable names that explain their purpose
3. Add meaningful comments and documentation
- Document why something is done, not what is being done
- Write docstrings for functions explaining parameters and return values
- Keep comments up-to-date with code changes
4. Keep functions small and focused
- Aim for functions that do one thing well
- Consider splitting functions longer than 20-30 lines
- Use clear return values and avoid side effects
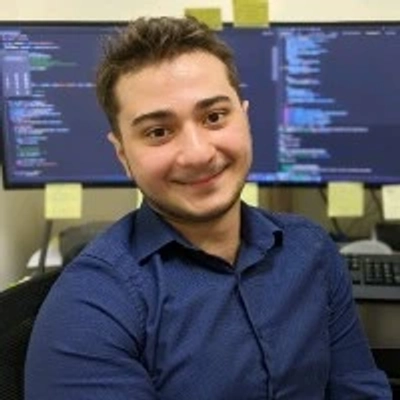
Adopt Modular Programming
Adopting modular programming has been the most valuable practice for writing cleaner, more maintainable code. By breaking functionality into smaller, reusable functions or classes, each handling a specific task, the code becomes easier to read, test, and debug. For example, while building a web app, I separated API calls, data processing, and UI rendering into distinct modules. This made updates or fixes simple since changes in one area didn't ripple across the entire codebase. Modular programming has improved my development process by promoting scalability, reducing duplication, and making collaboration with team members much smoother. Keep it modular-it's a game-changer!
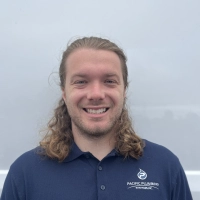
Emphasize Composability and Modularity
The best shift in my coding work came from a concerted effort to emphasize composability and modularity - that is, from breaking big tasks into tiny, sharp tools. Like a craftsman who reaches for just the right chisel, I now build small bits of code that do one thing, and do them really well.
This matches what old Unix hands knew: small tools that work together beat big, tangled systems. When I split work into bite-sized chunks, bugs have fewer places to hide.
Key benefits I've seen:
* Faster bug fixes - small tools mean small fixes
* Better testing - each piece can be checked alone
* Easier teamwork - others can understand discrete chunks
* More reuse - sharp tools find new uses
Take a recent project: Instead of one big CRM system, we built:
- An efficient Svelte web UI for end-users to enter sales data and train deep learning models
- A PostgreSQL backend for the CRM data
- A prospecting database using Clickhouse and DuckDB
- A data acquisition system using a hybrid cloud deployment
Each piece stood alone. When bugs crept in, it's easy to know where to look. When needs change, parts can be swapped without breaking the whole - we're looking right now at replacing some of the pieces with an Elixir-backed system for concurrency; because we engineered with small, composable parts, it's easy to see where we can add that to our architecture.
The hardest part was learning to spot where to make the cuts - like a butcher finding the joints. But that skill grows with practice. Now it's second nature to ask: "Could this be smaller? Could these pieces work alone?"
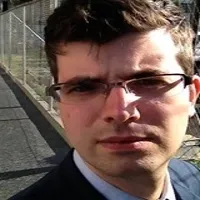
Implement Single Responsibility Principle
The most valuable coding practice that I've adopted for writing clean and maintainable code is the "Single Responsibility Principle." This is also one of the solid principles of object-oriented design. See how it improves the overall development process: When classes or modules are built to execute a single output, it becomes easy for other developers to understand the code without any mind-boggling. This enhances the readability of the entire code. This principle also simplifies the testing process with more straightforward unit tests to cover all the possible cases without complexity. The principle also helps in easier maintenance of code, and developers can modify the existing code without disturbing the functionality of the entire system. This also minimizes the chances of bugs during maintenance. The principle also helps in reusing the same classes repeatedly when required. This makes the entire code lightweight by minimizing extra lines of code needed to reuse the same class.
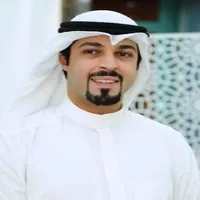
Write Small, Modular Functions
One of the most valuable coding practices I've adopted for writing cleaner and more maintainable code is the principle of writing small, modular functions. The idea is to break down complex tasks into smaller, more manageable pieces, each with a single responsibility. By doing this, I ensure that each function or method does one thing, and does it well, which not only improves readability but also makes it easier to test, debug, and extend.
This practice has had a huge impact on my development process. First, it reduces the cognitive load when revisiting or working with code I haven't seen in a while. Smaller functions are self-contained, meaning I can quickly understand their purpose and modify them without worrying about unintended side effects elsewhere in the codebase. It also helps with collaboration: when teammates work on the same project, they don't have to untangle large, monolithic functions; they can simply work on or adjust the relevant, isolated part of the code.
Additionally, this approach makes refactoring easier. Because the functions are modular, I can swap them out, extend them, or reuse them in different parts of the application with minimal effort. Over time, as the codebase grows, it becomes more scalable and less prone to bugs, which ultimately saves time and reduces technical debt. It's a simple practice, but one that has paid off hugely in terms of efficiency, clarity, and long-term maintainability.
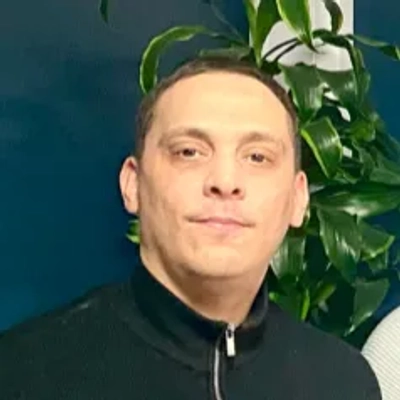
Write Clear Comments and Small Functions
The most valuable coding practice I've adopted is writing clear, descriptive comments and keeping functions small and focused. By breaking down complex tasks into smaller, manageable functions, it's easier to understand and modify the code later. Comments help others (and future me) quickly understand why certain decisions were made, which is crucial when you're working on a team or revisiting a project after some time. For example, in a recent project, we had to make frequent updates to a large codebase. Because I had kept functions concise and well-documented, the updates were quicker and less error-prone. This practice has saved a lot of time during code reviews and maintenance, making the development process more efficient overall.
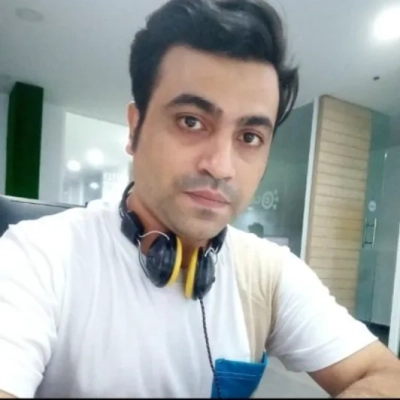